Python is among the most popular programming languages today. Major organizations in the world build programs and applications using this object-oriented language. Here, you will come across some of the most frequently asked questions about Python in job interviews in various fields. Python Topic Wise Interview Questions, Our Python interview questions for both experienced professionals and freshers will help you in your interview preparation. (Copied)
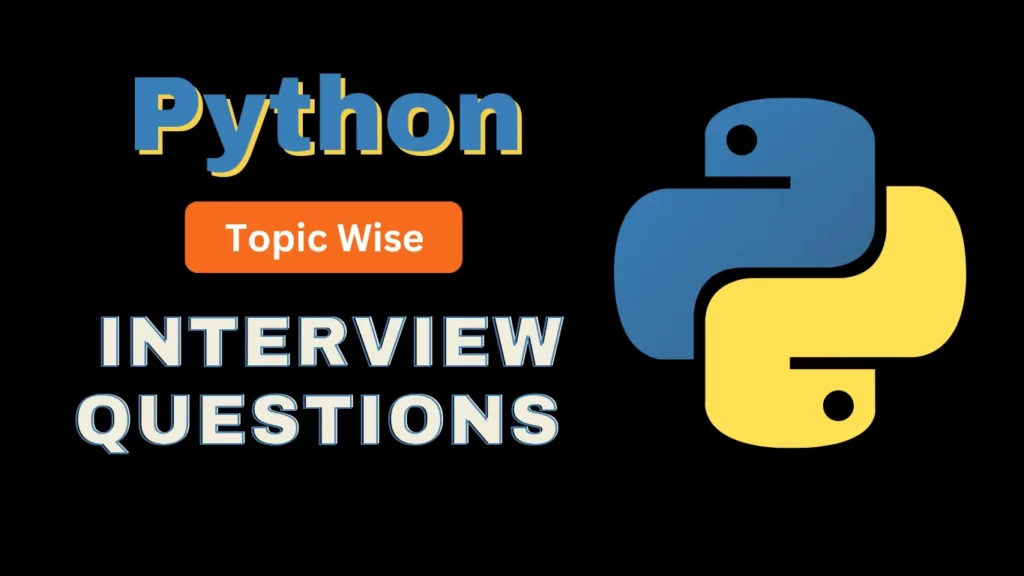
Python Interview Questions
1.Python Basics
Prepare for Python interviews with these essential basics. Find answers to common Python interview questions in this comprehensive guide.
Python Basics interview questions for freshers
- What is Python? Explain its key features.
- What is pep 8?
- How do you comment out a line of code in Python?
- What is the purpose of the “if name == ‘main’:” statement in Python?
- What is the difference between ‘==’ and ‘is’ in Python?
Python Basics interview questions for experienced
- Explain the concept of a generator in Python. How is it different from a normal function?
- How does exception handling work in Python? Give an example.
- What are decorators in Python? Provide an example of how they are used.
- What are the differences between shallow copy and deep copy in Python?
- How does Python’s garbage collection mechanism work?
Python Basics interview questions for experienced professionals
- Explain the Global Interpreter Lock (GIL) in Python. How does it impact multi-threading?
- What are metaclasses in Python? How and why would you use them?
- What is the purpose of the Python ‘yield’ keyword? Provide an example.
- Explain the concept of method resolution order (MRO) in Python.
- How do you optimize the performance of Python code? Provide some techniques.
2. Input/Output
Explore essential Python interview questions on Input/Output. Prepare for your next interview with this comprehensive guide.
Input/Output interview questions for freshers
- What is input and output in Python?
- How can you take user input in Python?
- How do you print output to the console in Python?
- Explain the difference between input() and raw_input() functions.
- How do you open a file in Python for reading and writing?
Input/Output interview questions for experienced
- What are the different modes in which a file can be opened in Python?
- How do you read a specific number of characters from a file in Python?
- Explain the difference between ‘w’ and ‘a’ modes when opening a file in Python.
- How can you read a CSV file in Python and extract data from it?
- How do you handle exceptions related to file input/output operations in Python?
Input/Output interview questions for experienced professionals
- What is the purpose of the “with” statement in Python file handling?
- How can you read and write binary files in Python?
- Explain the concept of file buffering and its significance in Python I/O.
- How do you redirect the standard output to a file in Python?
- What is the difference between text mode and binary mode in file handling?
3. Data Types
Explore essential Python interview questions related to data types. Prepare for your Python interviews with this comprehensive guide.
Data Types interview questions for freshers
- What are the built-in data types in Python?
- What is the difference between a list and a tuple?
- How do you define a dictionary in Python?
- What is the difference between a set and a frozenset?
- What is the purpose of the bool data type in Python?
- How do you check the data type of a variable in Python?
- How do you convert a string to an integer in Python?
- How do you convert a float to an integer in Python?
- What is the None data type used for in Python?
- How do you create an empty list in Python?
Data Types interview questions for experienced
- What is the difference between shallow copy and deep copy in Python?
- How do you convert a string to a list in Python?
- What are the different numeric data types available in Python?
- How do you convert a list of strings to a single string in Python?
- What are the differences between the append() and extend() methods in Python lists?
- How do you remove duplicate elements from a list in Python?
- Explain the concept of immutable data types in Python.
- How do you check if a key exists in a dictionary?
- What is the difference between a stack and a queue?
- What are the different methods available in Python sets?
Data Types interview questions for experienced professionals
- What are the differences between mutable and immutable data types?
- Explain the concept of iterators and generators in Python.
- How does garbage collection work in Python?
- How do you create a custom exception in Python?
- Explain the concept of namespaces in Python.
- What is the difference between a shallow copy and a deep copy in Python dictionaries?
- How do you implement a doubly linked list in Python?
- What is the purpose of the slots attribute in Python classes?
- How do you serialize and deserialize Python objects?
- Explain the concept of method resolution order (MRO) in Python.
4.Variables
Python interview questions on variables. Learn how they work and ace your next Python coding interview with confidence.
Variables interview questions for freshers
- What is a variable in Python?
- How do you define a variable in Python?
- What are the rules for naming a variable in Python?
- What is the difference between local and global variables?
- What is the purpose of the “None” value in Python?
- How do you assign a value to a variable in Python?
- How do you check the type of a variable in Python?
- What is variable scoping in Python?
- Can you reassign a different data type to a variable in Python?
- How do you delete a variable in Python?
Variables interview questions for experienced
- Explain the concept of pass-by-value and pass-by-reference in Python.
- What is variable unpacking in Python?
- How does garbage collection work for variables in Python?
- Discuss the difference between mutable and immutable variables in Python.
- What is variable shadowing in Python?
- Explain the concept of variable scope resolution in Python.
- How do you swap the values of two variables without using a temporary variable?
- What is the use of the “global” keyword in Python?
- Discuss the difference between “is” and “==” in Python when comparing variables.
- Explain the concept of variable interpolation in Python.
Variables interview questions for experienced professionals
- Describe the differences between the local, nonlocal, and global variable scope in Python.
- Explain the concept of closures in Python and how they relate to variables.
- What are the differences between instance variables and class variables in Python?
- Discuss the concept of garbage collection in Python and how it handles variables.
- How does Python handle memory management for variables?
- Explain the concept of variable annotations in Python.
- Describe the concept of variable hoisting and whether it applies in Python.
- Discuss the concept of variable scoping in list comprehensions in Python.
- How can you dynamically create variables in Python?
- Explain the concept of variable shadowing in the context of object-oriented programming in Python.
5.Operators
Operators interview questions for freshers
- What are operators in Python?
- What is the difference between arithmetic and assignment operators?
- Explain the concept of comparison operators in Python.
- How do you use logical operators in Python?
- Describe the purpose of the identity operators “is” and “is not.”
- What are membership operators in Python? Provide examples.
- How do you use the “not” operator in Python?
Operators interview questions for experienced
- What is operator precedence, and how is it determined in Python?
- Explain the ternary operator in Python and provide an example.
- What is the purpose of the bitwise operators in Python?
- How does short-circuiting work with logical operators in Python?
- Discuss the differences between “is” and “==” operators in Python.
- What are the in-place operators in Python? Provide examples.
- How do you overload operators in Python?
Operators interview questions for experienced professionals
- Explain the concept of operator overloading in Python.
- How can you define custom operators in Python?
- Discuss the use of magic methods for operator overloading in Python.
- What is the purpose of the “yield” keyword in Python? How is it related to operators?
- Explain the concept of operator precedence parsing in Python.
- How do you implement matrix multiplication using operator overloading in Python?
- Discuss the differences between Python’s “and” and “or” operators and their short-circuiting behavior.
6.Control Flow
Control Flow interview questions for freshers
- What is control flow in Python?
- What are the different types of control flow statements in Python?
- Explain the if statement in Python with an example.
- How do you use the for loop in Python?
- What is the purpose of the break statement in Python?
- Explain the while loop in Python with an example.
- How does the continue statement work in Python?
- What is the purpose of the pass statement in Python?
- How do you use the if-else statement in Python?
- Explain the use of the range() function in Python.
Control Flow interview questions for experienced
- What is the difference between while and do-while loops in Python?
- Explain the use of the elif statement in Python.
- How can you terminate a loop prematurely in Python?
- What are nested loops in Python? Provide an example.
- How do you use the try-except block for error handling in Python?
- Explain the use of the assert statement in Python.
- What are generator functions in Python? How are they different from normal functions?
- How can you use the else block with a loop in Python?
- Explain the concept of recursion in Python. Provide an example.
- What is the purpose of the finally block in Python exception handling?
Control Flow interview questions for experienced professionals
- What are decorators in Python? How can you use them to modify the behavior of functions?
- How does the context manager protocol work in Python?
- Explain the use of the zip() function in Python with an example.
- What is the purpose of the lambda function in Python? Provide a use case.
- How can you create a custom iterator in Python? Provide an example.
- Explain the concept of list comprehensions in Python.
- What are the differences between a shallow copy and a deep copy in Python?
- How can you handle multiple exceptions in Python using a single except block?
- Explain the concept of coroutine in Python.
- What are the differences between the range() and xrange() functions in Python 2?
7.Functions
Functions interview questions for freshers
- What is a function in Python?
- How do you define a function in Python?
- What is the purpose of the return statement in a function?
- What is the difference between a function definition and a function call?
- How do you pass arguments to a function in Python?
- What is the difference between positional and keyword arguments in Python?
- How do you handle default arguments in a function?
- What are the advantages of using functions in Python?
- How do you call a function recursively in Python?
- What is the difference between a built-in function and a user-defined function?
Functions interview questions for experienced
- What is variable scope in Python functions?
- What is a lambda function in Python? How is it different from a regular function?
- How do you pass a function as an argument to another function?
- What is the purpose of the *args and **kwargs parameters in a function?
- How do you write a function that accepts a variable number of arguments?
- What is function overloading? Does Python support function overloading?
- How do you handle exceptions in Python functions?
- Can you have multiple return statements in a function? If yes, what happens when the first return statement is encountered?
- What is the purpose of the global keyword in Python functions?
- How do you define a recursive function in Python?
- Explain the concept of “closure” in relation to lambda functions.
Functions interview questions for experienced professionals
- What is a closure in Python? How do you create a closure?
- How does the @staticmethod decorator work in Python functions?
- What are decorators in Python? How do they work?
- What is the difference between a generator function and a regular function?
- What is a coroutine in Python? How is it different from a regular function?
- What is the purpose of the yield keyword in a generator function?
- What are function annotations in Python? How do you use them?
- How do you create and use a generator expression in Python?
- How do you write a recursive function with memoization in Python?
- What are first-class functions in Python?
- How do you handle exceptions within a lambda function?
8.Object Oriented Concepts
Object Oriented Concepts interview questions for freshers
- What is Object-Oriented Programming (OOP)?
- What are the core principles of OOP?
- What is a class in Python?
- What is an object in Python?
- What is the difference between a class and an object?
- What is inheritance in OOP?
- What is encapsulation in Python?
- What is polymorphism in Python?
- What are the advantages of using OOP in Python?
- How do you create an instance of a class in Python?
Object Oriented Concepts interview questions for experienced
- What are the different access modifiers in Python?
- Explain the concept of method overriding in Python?
- What is the difference between composition and inheritance?
- How does Python support multiple inheritance?
- What are abstract classes and methods in Python?
- What is the difference between shallow copy and deep copy in Python?
Object Oriented Concepts interview questions for experienced professionals
- Explain the concept of method overloading in Python.
- What are static methods and class methods in Python?
- How does Python handle exceptions in an object-oriented program?
- What is the purpose of the super() function in Python?
- How do you implement operator overloading in Python?
- What are decorators in Python and how are they used in OOP?
- What is the difference between an instance variable and a class variable?
- Explain the concept of interfaces in Python.
- How do you implement data hiding in Python?
9. Exception Handling
Exception Handling interview questions for freshers
- What is exception handling in Python?
- How are exceptions raised and caught in Python?
- What is the purpose of the try-except block in Python?
- Explain the role of the else block in exception handling.
- What is the purpose of the finally block in exception handling?
Exception Handling interview questions for experienced
- What is the difference between a runtime error and a syntax error in Python?
- Explain the hierarchy of exception classes in Python.
- How can you handle multiple exceptions in a single except block?
- What is the purpose of the “raise” keyword in Python?
- How can you create custom exceptions in Python?
Exception Handling interview questions for experienced professionals
- Explain the difference between the “except” and “finally” blocks in Python.
- What are the best practices for using exception handling in Python?
- How can you handle and propagate exceptions in nested function calls?
- Explain the concept of exception chaining in Python.
- What are the differences between the “assert” statement and exception handling?
Python Collections
Python Collections interview questions for freshers
- What are the built-in collection types in Python?
- What is the difference between a list and a tuple?
- How do you access an element in a list or a tuple?
- What is a dictionary in Python?
- How do you add an element to a list?
- How do you remove an element from a list?
- How do you iterate over a dictionary?
Python Collections interview questions for experienced
- What is the difference between a set and a frozenset?
- How do you check if an element exists in a list or a dictionary?
- What are the main methods of a dictionary object?
- How do you sort a list in Python?
- Explain the concept of a default dictionary.
- How do you merge two dictionaries in Python?
- What is a deque and how is it different from a list?
Python Collections interview questions for experienced professionals
- What are the main differences between a list and a linked list?
- How do you implement a stack using a list or a deque?
- What is a heap and how is it implemented in Python?
- Explain the concept of a generator and provide an example.
- How do you implement a priority queue in Python?
- How do you implement a binary search tree in Python?
- What is the time complexity of common operations on different collection types?