In this post, we will learn about Python data types and how we can store the values in Python. As we already know about the storage of other programming languages like C, C++, Java etc where we use data types to store different types of data into variables. We are also aware of the data types like Integer, Float, Char, Boolean etc in other programming languages but we are not going to use such data types in Python. Confused! Yes, we are not using any data type in python for declaring variables. Let’s learn all data types one by one and learn about the variables also.
Variables
In programming languages, developers use variables to store data. Variables actually hold values of any type. Each variable must have a unique name called an identifier. It is helpful to think of variables as containers that hold data which can be changed later throughout programming.
Declaring Variables in Python
In Python, variables do not need a declaration to reserve memory space. The “variable declaration” happens automatically when we assign a value to a variable. Let’s declare some variables and assign the values.
a = 10
b = 5.4
c = "Hello World"
d = (1,2,3)
e, f, g = 10, 20.5, "Hello"
Above code snippet gives idea about assigning different values to the variables and declaring the variables. Some best practices while declaring the variables in python.
- Use Descriptive Variable Names
- Follow Python Naming Conventions like var_name instead of varName
- Avoid Single-Character Names
- Use Constants for Immutable Values
- Keep Variable Names Singular for Single Values
- Be Consistent with Naming Style
- Use Meaningful Prefixes for Specific Types
- Avoid Abbreviations and Acronyms
- Use Context-Appropriate Variable Names
- Refactor When Needed
Python Data Types
Basically data type represents the type of value and determines how the value can be used in a python program. Since all the data values are encapsulated in relevant object classes. Everything in Python, is simply an object and every object has an identity, a type and a value. The data stored in memory can be of any type. Below are the some high level data types in Python.
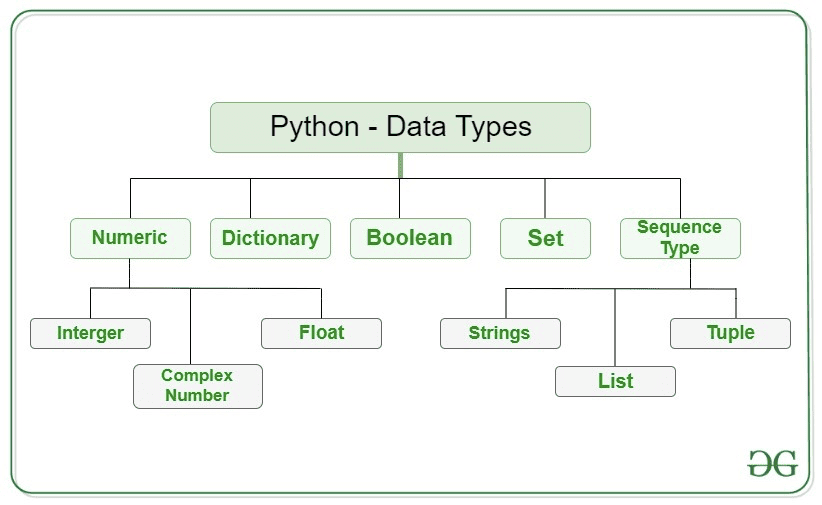
I hope, above figure shows all the important data types which we can use in Python. Let’s learn all the data types one by one.
Numeric : Python Data Types
In Python, numeric data type represent the data which has numeric value. Numeric value can be integer, floating number or even complex numbers. In Python, the int, float, and complex classes define these values. While declaring the variable, we don’t need to use this classes but when we assign the value to the variable it will automatically decided the data type in Python.
Integer
The int class represents an integer value. It contains positive or negative whole numbers (without fraction or decimal). In Python there is no limit to how long an integer value can be. Let’s declare the variable with Int type.
number_a = 10
number_b = 30
number_c = number_a + number_b
All above values are of Integer type and it’s result value is also of integer type. We can use type(number_a) function to get the data type class in Python. type(number_a) function will return the Int class.
Float
The float class represents an float value. It is a real number with floating point representation. It is specified by a decimal point. Optionally, the character e or E followed by a positive or negative integer may be appended to specify scientific notation. Let’s declare the variable with float type.
number_a = 10.30
number_b = 30
number_c = number_a + number_b
In above code, number_a is of Float type while number_b is of Integer type and it’s result value is float type. We can use type(number_a) function to get the data type class in Python. type(number_a) function will return the Float class.
Complex
The complex class represents an complex value. It is specified as (real part) + (imaginary part)j. For example – 2+3j. Number 2 is real while number with Character is imaginary. Let’s declare the variable with Complex type.
number_a = 2 + 3j
Above values are of Complex type and it’s result value will be also of complex type. We can use type(number_a) function to get the data type class in Python. type(number_a) function will return the Complex class.
Sequence : Python Data Types
In Python, a sequence is the ordered collection of similar or different data types. Sequences allow to store multiple values in an organised and efficient fashion. There are several sequence types in Python
- String
- List
- Tuple
String
As it’s name suggest, String can store the collection of characters in a contiguous order either in single quote or double quote. In python, we don’t need ‘String’ class to declare string variables like other programming language. There are multiple string operations which we can perform on string values but will see all those operations in separate post.
Subsets of strings can be taken using the slice operator ([ ] and [:] ) with indexes starting at 0 in the beginning of the string and working their way from -1 at the end.
The plus (+) sign is the string concatenation operator and the asterisk (*) is the repetition operator.
str_a = 'My Name is Alice'
str_b = "My Name is Bob"
Above both example or variables store the string value. We can use type(str_a) function to get the data type class in Python. The type(str_a) function will return the class ‘str’.
Below are the some operations on String value
str_a[ : : ] #will return whole string
str_a[ : : -1] #will return string in reverse order
str_a[ 0 : 3] #will return substring starting from 0 to 2
str_a[ 2 : 5] #will return substring starting from 2 to 4
str_a[ 4 : ] #will return substring starting from 4
str_a[6 : 4 : -1]. #will return substring starting from 6 to 5 in reverse order
print str_a * 2 #will print string twice
print str + "TEST" #will print string and append TEST
I hope, all above example clears the concept of slice operator. Here’s a selection of common string methods in Python:
Methods
-
len(str)
: Returns the length of the string. -
str.upper()
: Returns a copy of the string with all characters converted to uppercase. -
str.lower()
: Returns a copy of the string with all characters converted to lowercase. -
str.capitalize()
: Returns a copy of the string with the first character capitalized. -
str.title()
: Returns a copy of the string with the first character of each word capitalized. -
str.strip()
: Returns a copy of the string with leading and trailing whitespace removed. -
str.lstrip()
: Returns a copy of the string with leading whitespace removed. -
str.rstrip()
: Returns a copy of the string with trailing whitespace removed. -
str.startswith(prefix)
: ReturnsTrue
if the string starts with the specifiedprefix
. -
str.endswith(suffix)
: ReturnsTrue
if the string ends with the specifiedsuffix
. -
str.replace(old, new)
: Returns a copy of the string with all occurrences ofold
replaced bynew
. -
str.split(separator)
: Returns a list of substrings separated byseparator
. -
str.join(iterable)
: Concatenates elements of an iterable (e.g., tuple) into a string, using the string as a separator. -
str.find(sub)
: Returns the lowest index ofsub
in the string, or -1 if not found. -
str.count(sub)
: Returns the number of non-overlapping occurrences ofsub
in the string. -
str.isalnum()
: ReturnsTrue
if all characters in the string are alphanumeric (letters or digits). -
str.isalpha()
: ReturnsTrue
if all characters in the string are alphabetic. -
str.isdigit()
: ReturnsTrue
if all characters in the string are digits. -
str.islower()
: ReturnsTrue
if all cased characters in the string are lowercase. -
str.isupper()
: ReturnsTrue
if all cased characters in the string are uppercase. -
str.isnumeric()
: ReturnsTrue
if all characters in the string are numeric. -
str.isdecimal()
: ReturnsTrue
if all characters in the string are decimal characters. -
str.format()
: Formats the string by substituting placeholders with values. -
str.encode(encoding)
: Returns an encoded version of the string using the specified encoding.
These are just a few examples of the many string methods available in Python. Strings are a fundamental data type in Python, and understanding these methods can greatly enhance your ability to manipulate and process text data.
List
Lists are the most versatile of Python’s data types. A list contains items separated by commas and enclosed within square brackets ([]). To some extent, lists are similar to arrays in any programming language. One difference between them is that all the items belonging to a list can be of different data type while in other programming language it’s not allowed.
The values stored in a list can be accessed using the slice operator ([ ] and [:]) with indexes starting at 0 in the beginning of the list and working their way to end -1.
The plus (+) sign is the list concatenation operator, and the asterisk (*) is the repetition operator.
list_a = [1,2,3,4]
list_b = [1, "Hello", 3.4, True]
Above examples declare the list with similar or different data types. We can use type(list_a) function to get the data type class in Python. The type(list_a) function will return the List class.
Below are the some operations on List value
list_a = [ 1, 2, 3, 4, 5] #declare the list with 5 elements
list_a[2:4] #print the values from index 2 to 3
list_a + list_a #concatenate both lists
list_a * 2 #concatenate or repeat same list twice
These are the some list operations. Below are the list of some methods which are supported for List in Python.
Methods
-
list.append(item)
: Adds an item to the end of the list. -
list.extend(iterable)
: Appends elements from an iterable to the end of the list. -
list.insert(index, item)
: Inserts an item at the specified index. -
list.remove(item)
: Removes the first occurrence of the specified item. -
list.pop([index])
: Removes and returns the item at the specified index. If index is not provided, removes and returns the last item. -
list.index(item[, start[, end]])
: Returns the index of the first occurrence of the specified item in the list. -
list.count(item)
: Returns the number of occurrences of the specified item in the list. -
list.sort(key=None, reverse=False)
: Sorts the list in ascending order. Thekey
parameter can be used to specify a custom sorting function. -
list.reverse()
: Reverses the order of the list. -
list.copy()
: Returns a shallow copy of the list. -
list.clear()
: Removes all items from the list.
Lists are a fundamental and versatile data structure in Python.
Tuple
A tuple is another sequence data type that is similar to the list. A tuple consists of a number of values separated by commas. Unlike lists, however, tuples are enclosed within parentheses.The main differences between lists and tuples are: Lists are enclosed in brackets ( [ ] ) and their elements and size can be changed, while tuples are enclosed in parentheses ( ( ) ) and cannot be updated. Tuples can be thought of as read-only lists.
The values stored in a tuples can be accessed using the slice operator ([ ] and [:]) with indexes starting at 0 in the beginning of the list and working their way to end -1.
The plus (+) sign is the tuple concatenation operator, and the asterisk (*) is the repetition operator.
tuple_a = (1,2,3,4)
tuple_b = (1, "Hello", 3.4, True)
Above examples declare the tuple with similar or different data types. We can use type(tuple_a) function to get the data type class in Python. The type(tuple_a) function will return the Tuple class.
Below are the some operations on Tuple value
tuple_a = ( 1, 2, 3, 4, 5) #declare the tuples with 5 elements
tuple_a[2:4] #print the values from index 2 to 3
tuple_a + tuple_a #concatenate both tuples
tuple_a * 2 #concatenate or repeat same tuple_a twice
These are the some tuple operations. Below are the list of some methods which are supported for Tuple in Python.
Methods
-
tuple()
: Creates an empty tuple. -
tuple(iterable)
: Creates a tuple from an iterable, such as a list or another tuple. -
len(tuple)
: Returns the number of elements in the tuple. -
tuple.count(value)
: Returns the number of occurrences of a specified value in the tuple. -
tuple.index(value[, start[, end]])
: Returns the index of the first occurrence of a specified value. You can specify optionalstart
andend
parameters to search within a specific range. -
min(tuple)
: Returns the smallest element in the tuple. -
max(tuple)
: Returns the largest element in the tuple.
Tuples are particularly useful when you want to create collections of items that should not be modified after creation, or when you want to ensure data integrity. They are often used to represent related pieces of data, such as coordinates, database records, or multiple return values from functions.
Boolean : Python Data Types
Boolean data type store the values either True of False. This objects that are equal to True are truthy (true), and those equal to False are falsy (false). But non-Boolean objects can also undergo evaluation in a Boolean context, enabling us to determine whether they are true or false.
Note – True and False with capital ‘T’ and ‘F’ are valid booleans otherwise python will throw an error.
bool_a = True
bool_b = False
Above examples declare the boolean with True and False value. We can use type(bool_a) function to get the data type class in Python. The type(bool_a) function will return the Bool class.
Below are the some operations on Boolean value
bool_a = True
bool_a != True
bool_a == False
5 > 6
"lower".islower()
All above examples return the Boolean value either True or False. Some operators that you can use with Boolean values are
- Logical Operator
-
and
: ReturnsTrue
if both operands areTrue
. -
or
: ReturnsTrue
if at least one operand isTrue
. -
not
: Returns the opposite boolean value of the operand.
-
- Comparison Operator
-
==
: ReturnsTrue
if values are equal. -
!=
: ReturnsTrue
if values are not equal. -
<
: ReturnsTrue
if the left operand is less than the right operand. -
>
: ReturnsTrue
if the left operand is greater than the right operand. -
<=
: ReturnsTrue
if the left operand is less than or equal to the right operand. -
>=
: ReturnsTrue
if the left operand is greater than or equal to the right operand.
-
- Logical Expressions
- If
- elif
- else
- for
- while
Set : Python Data Types
In Python, Set is an unordered collection of data type that is iterable, mutable and has no duplicate elements. The order of elements in a set is undefined though it may consist of various elements.
Sets can be created by using the built-in set() function with an iterable object or a sequence by placing the sequence inside curly braces, separated by ‘comma’. Type of elements in a set need not be the same, various mixed-up data type values can also be passed to the set.
set1 = set()
set1 = set(["Geeks", "For", "Geeks"])
Above example declare the set with empty or the list of elements. We can pass the list to set for creating set of unique element. Set automatically remove the duplicate entries.
You cannot access set items by referring to an index because sets are unordered and do not have an index for their items. But you can loop through the set items using a for loop, or ask if a specified value is present in a set, by using the in keyword.
Below are the some operations on Set
set1 = set([1,2,3,4,5,6,6])
print(set1)
Presenting a list of sets’ common methods and operations that you can utilize:
Methods
-
set()
: Creates an empty set. -
set(iterable)
: Creates a set from an iterable (like a list, tuple, or string), removing duplicate elements. -
len(set)
: Returns the number of elements in the set. -
element in set
: Checks if an element is present in the set. -
element not in set
: Checks if an element is not present in the set. -
set.add(element)
: Adds an element to the set. -
set.remove(element)
: Removes a specified element from the set. Raises aKeyError
if the element is not found. -
set.discard(element)
: It removes a specified element from the set if it exists, without raising an error if the element is not found. -
set.pop()
: Removes and returns an arbitrary element from the set. Raises aKeyError
if the set is empty. -
set.union(other_set)
orset | other_set
: Returns a new set containing elements from both sets (no duplicates). -
set.intersection(other_set)
orset & other_set
: Returns a new set containing elements that are common to both sets. -
set.difference(other_set)
orset - other_set
: Returns a new set containing elements that are in the first set but not in the second set. -
set.symmetric_difference(other_set)
orset ^ other_set
: Returns a new set containing elements that are in either of the sets, but not in both. -
set.issubset(other_set)
: Verifies whether the set is a subset of another set. -
set.issuperset(other_set)
:Verifies whether the set is a superset of another set. -
set.intersection_update(other_set)
: Updates the set with the intersection of itself and another set. -
set.difference_update(other_set)
: Updates the set with the difference of itself and another set. -
set.symmetric_difference_update(other_set)
: Updates the set with the symmetric difference of itself and another set. -
set.copy()
: Returns a shallow copy of the set. -
set.clear()
: Removes all elements from the set
Sets are useful for tasks that involve handling unique elements, such as removing duplicates from a list, testing membership, and performing set operations like union, intersection, and difference.
Dictionary : Python Data Types
A dictionary is a built-in data type in Python that allows you to store and retrieve key-value pairs. Each key in a dictionary possesses uniqueness and serves as an access point for its corresponding value. Other programming languages refer to dictionaries as “associative arrays” or “hash maps.”
We can create a dictionary by enclosing key-value pairs within curly braces
{}
. Each key is separated from its value by a colon
:
. Keys must be unique and immutable (strings, numbers, or tuples), while values can be of any data type.
# Creating a dictionary
my_dict = {
"name": "John",
"age": 30,
"city": "New York"
}
You can access the values of a dictionary by using the keys as the index. If the key is not found, it raises a
KeyError
. You can also use the
get()
method to retrieve values, which allows you to provide a default value if the key is not found.
We can add new key-value pairs to a dictionary, and we can also remove items using the
del
statement or the
pop()
method.
# Adding and removing items
my_dict["occupation"] = "Engineer" # Adding a new key-value pair
del my_dict["city"] # Removing the "city" key
my_dict.pop("age") # Removing "age" using pop()
Python dictionaries provide various methods to manipulate and access their data
Methods
-
keys()
: Returns a list of all the keys in the dictionary. -
values()
: Returns a list of all the values in the dictionary. -
items()
: Returns a list of key-value pairs as tuples. -
clear()
: Removes all items from the dictionary. -
copy()
: Returns a shallow copy of the dictionary. -
update(other_dict)
: Updates the dictionary with key-value pairs from another dictionary.
Some operations on Python dictionary
keys_list = my_dict.keys()
values_list = my_dict.values()
items_list = my_dict.items()
# Modifying using update()
new_data = {"country": "USA", "occupation": "Software Engineer"}
my_dict.update(new_data)
Dictionaries are a powerful and versatile data structure in Python, allowing you to efficiently organise and retrieve data based on meaningful keys.
I hope all above sections clears the concepts of Python data types. There are some more concepts are related to data types or variables which we can cover in next session. To learn about the SQL, follow SQL Tutorials. Happy offline learning… Lets meet in next session.